Objectives
- To practice JavaScript, focusing on functions and "event driven"
programming.
- To review HTML and CSS.
Overview
For this project you will implement a webpage that allows the user
to play the game Rock-Paper-Scissors against the computer. The
computer's moves are chosen randomly.
How to Play Rock-Paper-Scissors
This is a classic game that is over 2000 years old. Two players face each other. After saying out loud "Rock, Paper, Scissors", the
players will simultanously hold out their hand showing one of the three gestures below:
The rules are simple:
- If both players show the same gesture, then it is a tie. Otherwise:
- Rock beats Scissors
- Paper beats Rock
- Scissors beats Paper
Demonstration
Here is a video that demonstrates what your webpage should look like and how it will work. Please note
that this video has sound (I recorded myself talking) so be sure to have your sound on.
Below is a screenshot of the program, for your reference:
Images
To begin this project, please download the following zip file. Unzip it. You will find a
folder called
p4, which contains the 9 images you see below. Be sure to put your proj4.html and proj4.css files into this same folder.
rock.jpg |  |
paper.jpg |  |
scissors.jpg |  |
leftRockHand.jpg |  |
leftPaperHand.jpg |  |
leftScissorHand.jpg |  |
rightRockHand.jpg |  |
rightPaperHand.jpg | 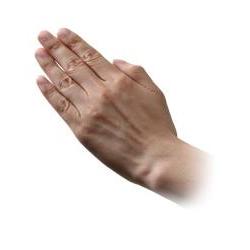 |
rightScissorHand.jpg |  |
Warning
This project requires a significant amount of time. Please start working on
the project early. Office hours get busy during the few days before
the project is due, and you may not get the help
you need if you wait too long. As always, we will not grant project extensions due to
technical problems on your end.
Hints:
- The whole thing can be rendered as just a single table. The table will have 5 rows and 3 columns.
You will need to make heavy use of rowspan and
colspan. For example, the entry at the top that says "Rock Paper Scissors" spans all three columns.
Another example: The boxes that show the players' hands -- each of these spans 3 rows.
- Be sure to use identifiers for elements that will change while the program is running.
- You will need to use the onClick attribute for the three elements that the user will click on.
I suggest having all three of these call the same function. This works well if you use a parameter
that represents whichever gesture has been selected. For example, I wrote a function called playGame that
has a parameter. When the user clicks the rock icon, I call it this way: playGame("rock"). When the
user clicks the paper icon, I call it this way: playGame("paper"). Same idea for scissors.
- Your javascript should probably use 5 variables because there are 5 things that change while the program is running.
Here are the ones I used and their starting values:
- wins = 0;
- losses = 0;
- ties = 0;
- picForLeftPlayer = "leftPaperHand.jpg";
- picForRightPlayer = "rightRockHand.jpg";
- To determine a random move for the opponent, you can call the random number generator like this: value = Math.random();.
Recall that the value will be a number between 0 and 1. If the value is smaller than 0.3333, use rock. If it is between 0.3333 and
0.6666 use paper, and if it is larger than 0.6666 go with scissors.
Requirements:
- You must create two files, named: proj4.html and proj4.css. The
HTML file will link to the CSS file, as usual.
- Your webpage must look exactly like the screenshot above, and must
behave as illustrated by the video.
- Your project should work properly for any sequence of moves the player makes (not just
the particular sequence of moves that I happened to demonstrate in the video). The player
can choose any of the three gesetures at any time, and can play for as long as he/she wants to.
- Please have the program begin by displaying "Paper" for the player on the left
and "Rock" for the opponent.
- The only font you may use is arial.
- Elements must be aligned in the same way we have them. In particular, the table should be centered horizontally within
the browser.
- The background color for the whole page must be green.
- Both your html and your CSS must validate according to these validators:
- Be sure to test your project with the Chrome browser -- that is how we will test it.
- You may not use any authoring tool (Dreamweaver, Frontpage, etc.) that generates the HTML for you.
- You must implement this project by yourself.
- DO NOT POST YOUR SOLUTION TO THIS PROJECT ON ANY WEB SERVER.
Submission
To submit your project, follow these steps:
- Create a zip file called p4.zip that includes 11 files:
- proj4.html
- proj4.css
- 9 jpg files (the images that were given to you)
- Upload the zip file using the submit server available at: https://submit.cs.umd.edu/.
Make sure you select the submit server entry (Project #4) that
corresponds to this project.
- After submitting your project, make sure you download from the submit
server the submitted file and verify that what you have submitted is
correct. Important: you must uncompress (extract) what you download
from the submit server, otherwise it may not be rendered correctly.
We will not accept projects sent via e-mail.
Academic Integrity
Please make sure you read the academic integrity section of the syllabus
so you understand what is permissible in our projects. We want to remind you
that we check your project against other students' projects and any case of
academic dishonesty will be referred to the University's Office of Student Conduct.
Web Accessibility